Why Golang?
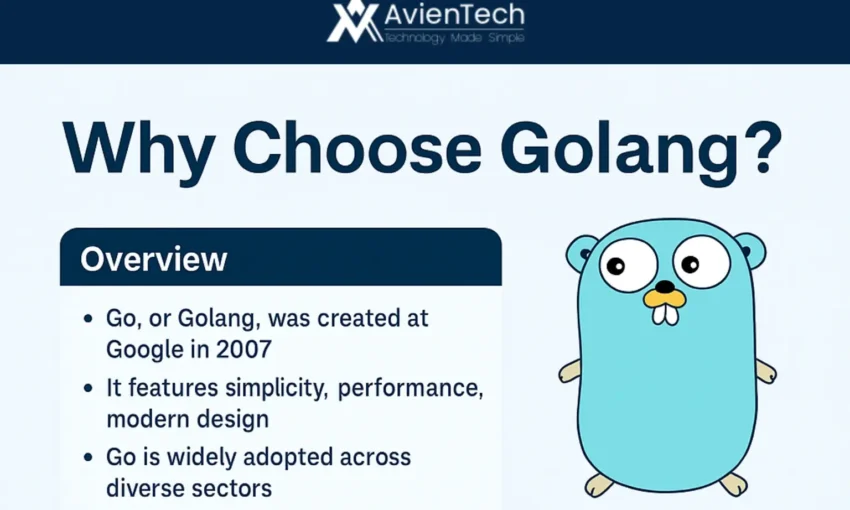
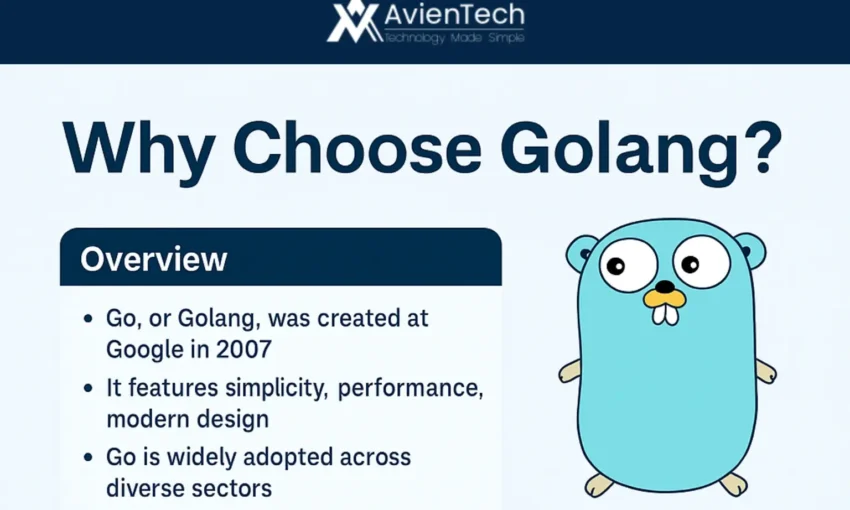
Go (or Golang) has emerged as one of the most significant programming languages in modern software development since its creation at Google in 2007. Its combination of simplicity, performance, and modern design principles has led to widespread adoption across diverse sectors. Let’s explore what makes Go special and why you might want to consider it for your next project.
The Origins of Go
Go was created by Robert Griesemer, Rob Pike, and Ken Thompson at Google, with its first public release in 2009. These creators designed Go to address common frustrations they experienced with existing languages while maintaining the strengths that made those languages successful.
Key Features That Make Go Stand Out
Simplicity and Readability
Go’s syntax is deliberately minimal and clear. With only 25 keywords, Go (or Golang) prioritizes readability and ease of learning. This simplicity reduces the cognitive load on developers and makes codebases more maintainable.
goCopypackage main
import "fmt"
func main() {
fmt.Println("Hello, Go!")
}
Built-in Concurrency
Go’s goroutines and channels provide elegant solutions for concurrent programming:
goCopyfunc main() {
// Create a channel
messages := make(chan string)
// Start a goroutine
go func() {
messages <- "Hello from another goroutine!"
}()
// Receive the message
msg := <-messages
fmt.Println(msg)
}
Fast Compilation
Go compiles directly to machine code with remarkable speed. This quick feedback loop enhances developer productivity and workflow efficiency.
Robust Standard Library
Go ships with a comprehensive standard library that covers networking, cryptography, compression, and more—reducing dependency on third-party packages.
Static Typing with Type Inference
Go combines the safety of static typing with the convenience of type inference, striking a balance between strictness and developer comfort.
Real-World Applications
Cloud Services and Microservices
Go has become the backbone of cloud infrastructure, with projects like Docker, Kubernetes, and Prometheus all written in Go. Its efficient resource usage and quick startup times make it ideal for containerized environments.
Web Development
Go’s http package makes building web servers straightforward, while frameworks like Gin and Echo provide additional conveniences for API development.
DevOps and Tooling
Go’s ability to compile to a single binary without dependencies makes it perfect for CLI tools and DevOps automation.
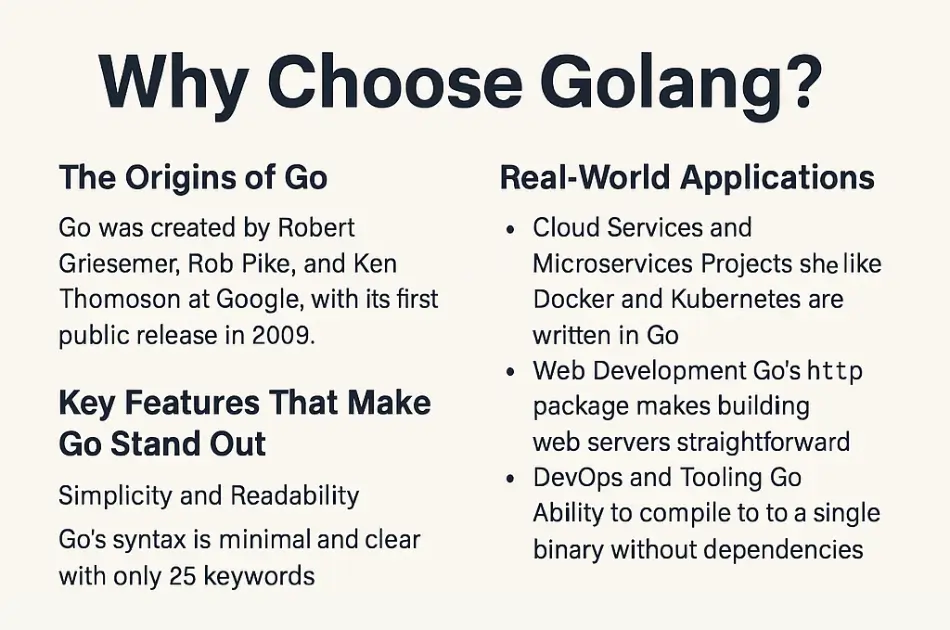
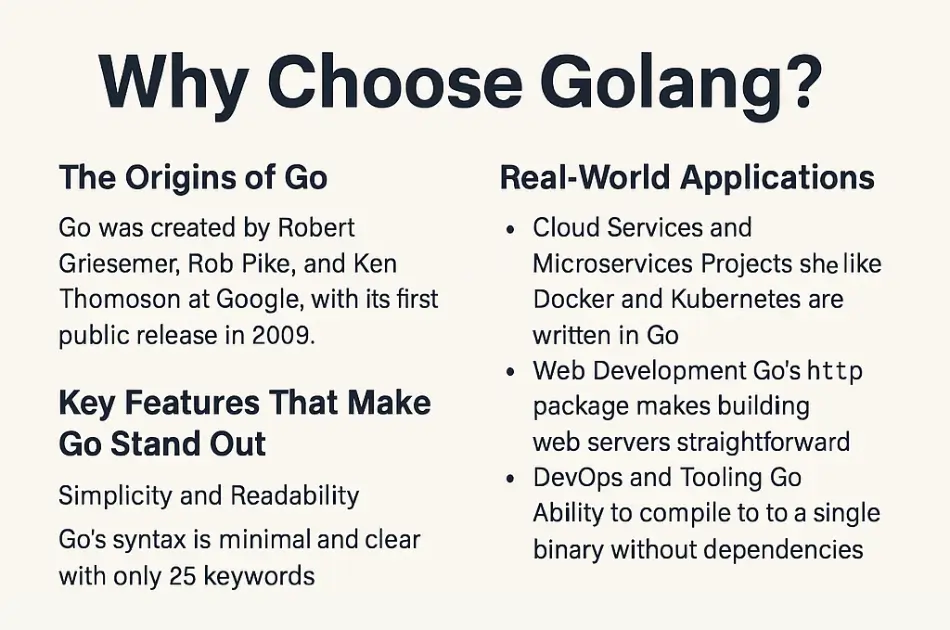
Companies Using Go (or Golang)
Many industry leaders have adopted Go, including:
- Google (where Go originated)
- Uber
- Twitch
- Dropbox
- PayPal
- American Express
Cons to Consider
Lack of Generics (Historic Issue)
Until Go 1.18 (released in March 2022), the lack of generics was often cited as a limitation. With their introduction, this criticism has been largely addressed.
Verbose Error Handling
Go’s explicit error handling can be repetitive:
goCopyresult, err := someFunction()
if err != nil {
// Handle error
return err
}
// Use result
Is Go Right for Your Next Project?
Consider Go when you need:
- High-performance network services
- Scalable concurrent applications
- Efficient resource utilization
- Fast development cycles
Go might not be ideal for:
- GUI desktop applications
- Complex mathematical computations
- Heavily object-oriented designs
Conclusion of Golang
Go’s focus on simplicity, performance, and practical functionality has earned it a prominent place in modern software development. Whether you’re building microservices, command-line tools, or web applications, Go offers a productive and reliable platform. Its thoughtful design choices reflect a philosophy that values clarity and maintainability—qualities that become increasingly important as projects grow.
By learning Go (or Golang), you’re not just picking up another programming language; you’re embracing a different way of thinking about software development that prioritizes straightforward solutions to complex problems.
Frequently Asked Questions about Why Choose Golang?
1. Is Go difficult to learn for beginners?
Go is considered one of the easier programming languages to learn, especially for developers with prior experience. Its minimalist syntax, comprehensive documentation, and straightforward approach to problem-solving make it accessible for beginners. The official Go tour and extensive community resources help newcomers get up to speed quickly.
2. How does Go’s performance compare to other languages?
Go offers performance that approaches lower-level languages like C and C++ while maintaining the development speed of higher-level languages. It achieves this through direct compilation to machine code, efficient garbage collection, and a runtime designed for concurrent execution. For most web services and networked applications, Go provides excellent performance with significantly less complexity than C/C++.
3. What makes Go especially good for cloud and microservices?
Go excels in cloud environments for several reasons: its small memory footprint, quick startup times, built-in concurrency, and compilation to standalone binaries. These characteristics make Go applications ideal for containerization and microservice architectures where resources must be used efficiently and services need to scale horizontally.
4. How mature is the Go ecosystem?
Despite being relatively young compared to languages like Java or Python, Go’s ecosystem has matured rapidly. The standard library is robust, covering most common needs. The package ecosystem continues to grow, with well-maintained libraries for web development, database access, message queuing, and more. The introduction of modules in Go 1.11 improved dependency management significantly.
5. Can Go replace Java or Python in enterprise environments?
Go is increasingly being adopted in enterprise environments, though it often complements rather than fully replaces established languages. Many companies use Go for specific components where its strengths in performance and concurrency are most valuable, while maintaining existing codebases in other languages. Go’s simplicity and maintainability make it particularly attractive for services that need to be maintained long-term by changing teams.