Mastering Loops in Golang
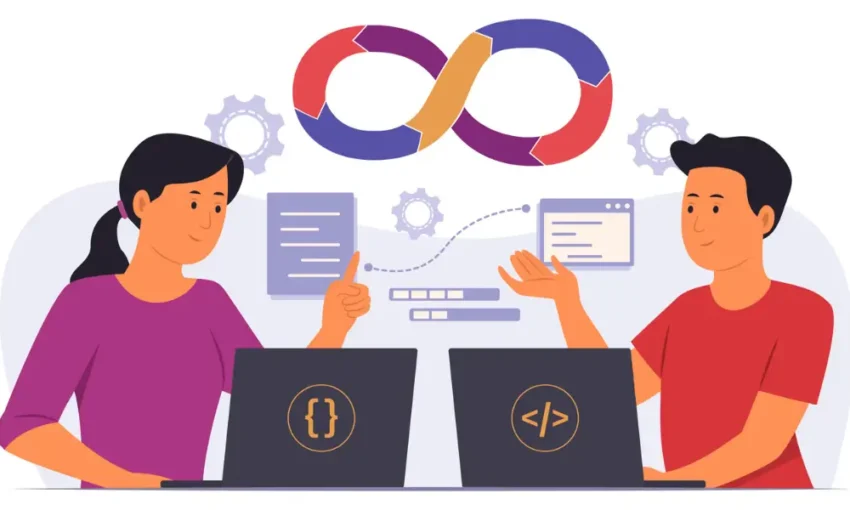
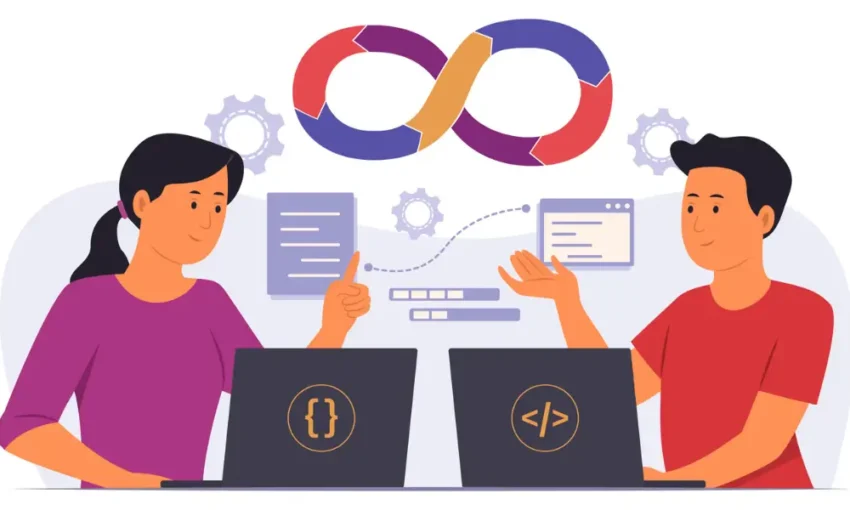
Loops are fundamental building blocks in Go programming, enabling developers to iterate through data structures and execute repetitive tasks efficiently. In this comprehensive guide, we’ll explore everything you need to know about loops in Golang, from basic syntax to advanced implementation strategies.
Understanding the Three Main Types of Loops in Go
Go provides three primary ways to implement loops, making it more straightforward than many other programming languages. Let’s dive deep into each type and understand their practical applications.
1. The Classic For Loop
The traditional for loop in Go follows a simple yet powerful syntax that consists of three components: initialization, condition, and post statement. Here’s how it works:
goCopyfor i := 0; i < 5; i++ {
fmt.Println(i)
}
This structure is perfect for when you need to:
- Execute code a specific number of times
- Iterate through array indices
- Implement counters with custom increments
2. The While-Style For Loop
Unlike other languages, Go doesn’t have a dedicated while keyword. Instead, it uses the for keyword with a condition:
goCopycount := 0
for count < 5 {
fmt.Println(count)
count++
}
This format is ideal when:
- The number of iterations is unknown
- You need to check a condition before each iteration
- Working with user input or external data
3. The Infinite Loop
Go makes it easy to create infinite loops when needed:
goCopyfor {
fmt.Println("This will run forever")
// Use break to exit the loop
}
Advanced Loop Patterns and Best Practices
Range-based Loops
One of Go’s most powerful features is the range keyword, which simplifies iteration over various data structures:
goCopy// Iterating over slices
fruits := []string{"apple", "banana", "orange"}
for index, value := range fruits {
fmt.Printf("Index: %d, Value: %s\n", index, value)
}
// Iterating over maps
scores := map[string]int{"Alice": 95, "Bob": 88}
for key, value := range scores {
fmt.Printf("%s scored %d\n", key, value)
}
Loop Control Statements
Go provides several statements to control loop execution:
- break – Exits the loop immediately
- continue – Skips to the next iteration
- goto – Transfers control to a labeled statement
Performance Optimization Tips
To write efficient loops in Go:
- Avoid unnecessary memory allocation inside loops
- Use proper data structures for iteration
- Consider using parallel processing for large datasets
- Cache length calculations outside the loop
goCopy// Inefficient
for i := 0; i < len(slice); i++ {
// Length calculated each iteration
}
// Efficient
length := len(slice)
for i := 0; i < length; i++ {
// Length calculated once
}
Common Use Cases and Examples
Working with Files
goCopyfile, err := os.Open("data.txt")
if err != nil {
log.Fatal(err)
}
defer file.Close()
scanner := bufio.NewScanner(file)
for scanner.Scan() {
fmt.Println(scanner.Text())
}
Processing API Responses
goCopyfor _, item := range apiResponse.Items {
if item.Status == "active" {
processItem(item)
}
}
Troubleshooting Common Loop Issues
- Infinite Loop Prevention
- Always ensure your loop has a clear exit condition
- Include safety checks for maximum iterations
- Implement timeout mechanisms for critical sections
- Memory Management
- Release resources properly within loops
- Use defer statements when appropriate
- Be cautious with goroutines in loops
Conclusion
Mastering loops in Golang is essential for writing efficient and maintainable code. Whether you’re working with simple iterations or complex data processing, understanding the various loop types and their optimal use cases will significantly improve your Go programming skills.
Remember to always consider performance implications and choose the most appropriate loop structure for your specific use case. With practice and proper implementation, you’ll be able to write more efficient and cleaner Go code.