Implementing Floyd’s Cycle-Finding Algorithm
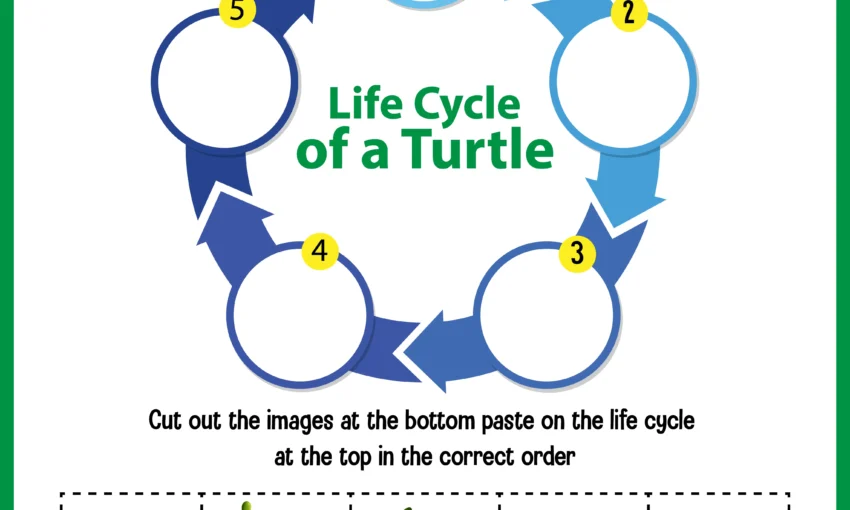
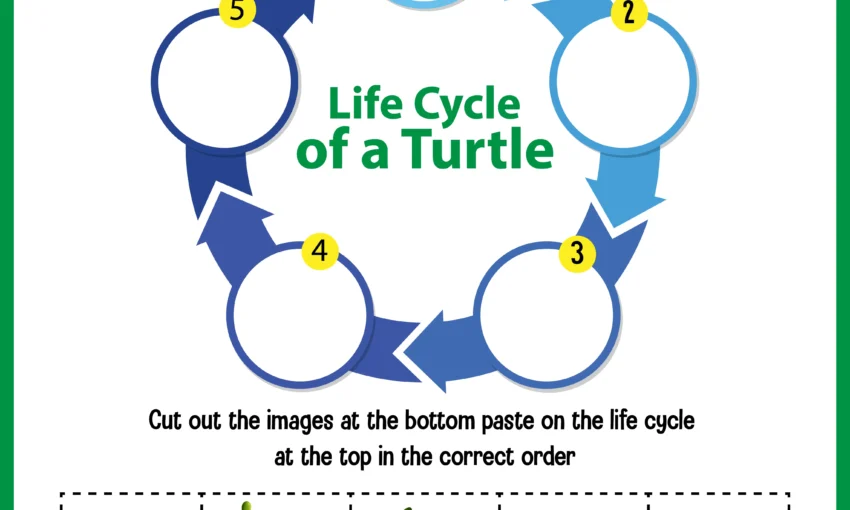
Floyd’s Cycle-Finding Algorithm, also known as the “tortoise and hare” algorithm, is a highly efficient technique for detecting cycles in sequences. In this comprehensive guide, we’ll explore how to implement this powerful algorithm and understand its practical applications in software development.
What is Floyd’s Cycle-Finding Algorithm?
Floyd’s Cycle-Finding Algorithm is an elegant solution for detecting cycles in linked lists or sequences. Developed by Robert W. Floyd, this algorithm uses two pointers moving at different speeds to determine if a cycle exists and find the start of the cycle.
Key Components of the Algorithm
1. The Two-Pointer Approach
The algorithm employs two pointers:
- The “tortoise” pointer moves one step at a time
- The “hare” pointer moves two steps at a time
When these pointers meet, it confirms the presence of a cycle in the sequence.
2. Implementation Steps
pythonCopydef detectCycle(head):
# Initialize pointers
tortoise = hare = head
# Phase 1: Detecting the cycle
while hare and hare.next:
tortoise = tortoise.next
hare = hare.next.next
if tortoise == hare:
return True
return False
3. Finding the Cycle Start Point
Once a cycle is detected, finding its starting point involves:
- Resetting one pointer to the head
- Moving both pointers at the same speed
- The meeting point indicates the cycle’s start
Practical Applications
1. Memory Leak Detection
- Identifying circular references in memory management
- Detecting infinite loops in program execution
2. Data Structure Validation
- Verifying linked list integrity
- Checking for cycles in directed graphs
3. Performance Optimization
- Space-efficient cycle detection (O(1) space complexity)
- Time-efficient algorithm (O(n) time complexity)
Implementation Best Practices
- Error Handling
- Always check for null pointers
- Handle edge cases (empty lists, single-node lists)
- Performance Considerations
- Avoid unnecessary memory allocation
- Implement optimal pointer movement
- Code Organization
- Separate cycle detection and finding cycle start
- Maintain clean, readable code structure
Common Pitfalls and Solutions
- Infinite Loops
- Always include proper termination conditions
- Verify pointer movement logic
- Memory Management
- Ensure proper pointer initialization
- Handle memory deallocation in non-garbage-collected languages
- Edge Cases
- Test with various input sizes
- Consider special cases like self-loops
Real-World Applications
- Database Systems
- Detecting circular dependencies
- Validating referential integrity
- Network Protocols
- Finding routing loops
- Detecting circular references in distributed systems
- Software Testing
- Identifying infinite recursion
- Validating data structure integrity
Optimization Techniques
- Space Optimization
- Minimize variable usage
- Utilize in-place operations
- Time Optimization
- Reduce unnecessary comparisons
- Implement efficient pointer movement
Conclusion
Floyd’s Cycle-Finding Algorithm remains a fundamental tool in computer science, offering an elegant solution to cycle detection problems. Its implementation requires careful attention to detail but rewards developers with an efficient and reliable method for handling cyclic structures in their applications.
Frequently Asked Questions (FAQ):
What is the time complexity of Floyd’s Cycle-Finding Algorithm?
The algorithm runs in O(n) time complexity, where n is the number of nodes in the linked list. The space complexity is O(1) as it only uses two pointers regardless of input size.
Can Floyd’s Algorithm detect cycles in any type of linked list?
Yes, the algorithm works with singly linked lists, doubly linked lists, and even sequences in memory. It’s versatile enough to handle various data structures where cycle detection is needed.
How does Floyd’s Algorithm differ from other cycle detection methods?
Unlike hash table-based methods that require O(n) space, Floyd’s Algorithm uses constant space. It’s more memory-efficient than alternatives while maintaining linear time complexity.
Is Floyd’s Algorithm suitable for detecting cycles in large datasets?
Yes, the algorithm is highly scalable and performs well with large datasets due to its linear time complexity and constant space usage.
Can Floyd’s Algorithm find the length of a cycle?
Yes, once the cycle is detected, you can count the steps needed for one pointer to complete one full cycle while keeping the other pointer stationary.