How to Use Event Aggregator in Aurelia
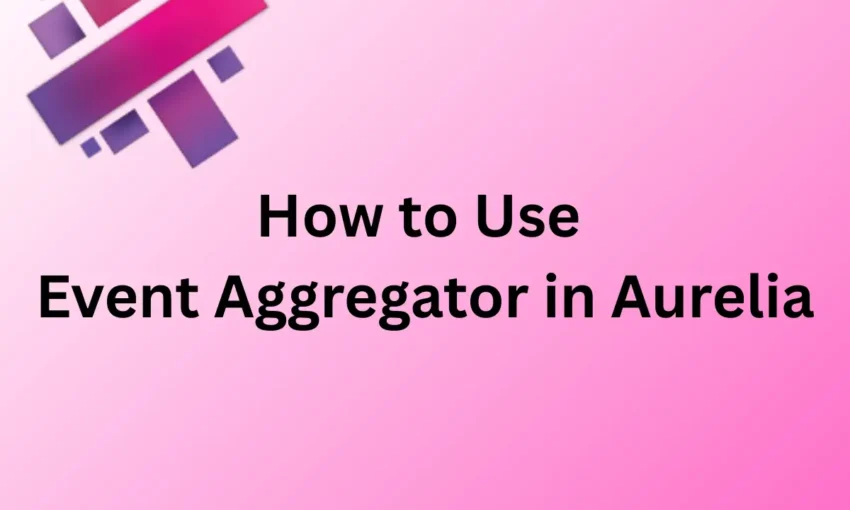
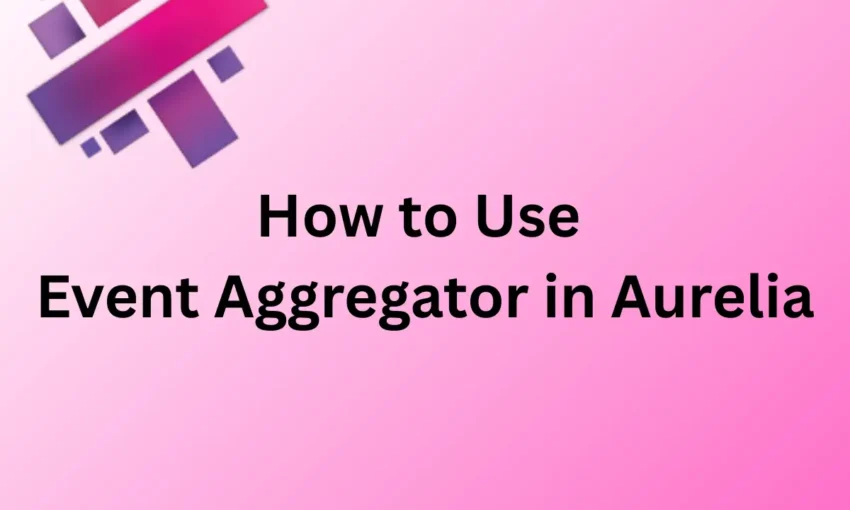
The Event Aggregator is a powerful feature in Aurelia that allows components to communicate with each other without direct coupling. This pattern is especially useful in large applications where components need to share information but maintaining clean architecture is essential. Let’s explore how to effectively implement and use the Event Aggregator in your Aurelia applications.
What is the Event Aggregator?
The Event Aggregator is a publish-subscribe messaging system that enables different parts of your application to communicate without having direct references to each other. This pattern helps you create loosely coupled components, making your code more maintainable and testable.
Setting Up Event Aggregator in Aurelia
Installation
If you’re using Aurelia 2, the Event Aggregator is available as a separate package:
npm install @aurelia/event-aggregator
For Aurelia 1, it’s included in the core framework.
Configuring the Event Aggregator
In your Aurelia application, you need to register the Event Aggregator as a dependency:
In Aurelia 2:
import { EventAggregator } from '@aurelia/event-aggregator';
export class MyApp {
static dependencies = [EventAggregator];
}
In Aurelia 1:
import { PLATFORM } from 'aurelia-framework';
export function configure(aurelia) {
aurelia.use
.standardConfiguration()
.developmentLogging();
aurelia.start().then(() => aurelia.setRoot(PLATFORM.moduleName('app')));
}
Using the Event Aggregator
Step 1: Inject the Event Aggregator
First, inject the Event Aggregator into your component or service:
import { EventAggregator } from 'aurelia-event-aggregator'; // Aurelia 1
// OR
import { EventAggregator } from '@aurelia/event-aggregator'; // Aurelia 2
export class MyComponent {
static inject = [EventAggregator]; // Aurelia 1
constructor(eventAggregator) {
this.ea = eventAggregator;
}
}
Step 2: Publishing Events
To publish an event, use the publish
method:
export class Publisher {
static inject = [EventAggregator];
constructor(eventAggregator) {
this.ea = eventAggregator;
}
sendMessage() {
this.ea.publish('message-channel', { content: 'Hello World', timestamp: new Date() });
}
}
Step 3: Subscribing to Events
To receive events, use the subscribe
method:
export class Subscriber {
static inject = [EventAggregator];
constructor(eventAggregator) {
this.ea = eventAggregator;
this.subscription = null;
this.messages = [];
}
attached() {
this.subscription = this.ea.subscribe('message-channel', message => {
this.messages.push(message);
console.log('Received message:', message.content);
});
}
detached() {
// Important: Always dispose of subscriptions when no longer needed
if (this.subscription) {
this.subscription.dispose();
}
}
}
Advanced Event Aggregator Techniques
Using Event Classes
For more complex applications, you can create custom event classes:
export class UserLoggedInEvent {
constructor(user) {
this.user = user;
}
}
// Publishing with event class
this.ea.publish(UserLoggedInEvent, new UserLoggedInEvent(user));
// Subscribing to event class
this.ea.subscribe(UserLoggedInEvent, event => {
console.log('User logged in:', event.user.name);
});
Using Event Handlers Decorator
Aurelia provides a convenient way to handle events using decorators (in Aurelia 1):
import { eventHandler } from 'aurelia-event-aggregator';
export class Dashboard {
@eventHandler(UserLoggedInEvent)
handleUserLogin(event) {
this.currentUser = event.user;
}
}
One-Time Events with subscribeOnce
If you only need to listen for an event once:
this.ea.subscribeOnce('app-initialized', () => {
this.performOneTimeSetup();
});
Best Practices for Using Event Aggregator
1. Clean Up Subscriptions
Always dispose of subscriptions when a component is detached or destroyed:
detached() {
this.subscription.dispose();
}
2. Use Meaningful Channel Names
Choose descriptive channel names to make your code more maintainable:
// Bad
this.ea.publish('channel1', data);
// Good
this.ea.publish('user:profile-updated', userData);
3. Document Your Events
Keep documentation of all events used in your application to help your team understand the communication flow:
/**
* Event: user:profile-updated
* Published when: User updates their profile information
* Data structure: { id: string, name: string, email: string }
* Subscribers: UserProfileView, HeaderComponent
*/
4. Consider Event Payload Structure
Keep event payloads consistent and focused:
// Good approach
this.ea.publish('order:created', {
orderId: 'ORD123',
timestamp: new Date(),
total: 59.99
});
Common Use Cases for Event Aggregator
- Notifications: Display notifications or alerts across the application
- Authentication State: Broadcast login/logout events
- Data Refresh: Notify components when data has been updated
- Modal Communication: Inform other components when modals are opened or closed
- Application State Changes: Broadcast important state changes
Troubleshooting Event Aggregator Issues
Events Not Being Received
If subscribers aren’t receiving events:
- Check that the event name/channel is identical in both publisher and subscriber
- Verify that the subscription is created before the event is published
- Ensure the subscription hasn’t been disposed
Memory Leaks
If you notice memory issues:
- Always dispose of subscriptions in
detached
or equivalent lifecycle methods - Use WeakMap for storing subscription references if needed
- Consider using
subscribeOnce
for one-time events
Conclusion
The Event Aggregator pattern in Aurelia provides a clean and efficient way for components to communicate without tight coupling. By following the best practices outlined in this guide, you can create more maintainable and scalable applications with clear communication patterns.
When used correctly, Event Aggregator can significantly improve the architecture of your Aurelia applications by reducing dependencies between components and making your code more modular. Start implementing this pattern today to experience the benefits of loosely coupled communication in your applications.
FAQs
1. What is an Event Aggregator in Aurelia?
The Event Aggregator in Aurelia is like a messaging system. It lets different sections of your app talk to each other without needing to know one another directly. This keeps everything flexible since components can share events that others can pick up on and respond to.
2. How do I install the Event Aggregator in Aurelia?
In Aurelia 2, you can set up the Event Aggregator by running this command: npm install @aurelia/event-aggregator
. If you’re using Aurelia 1, it’s already part of the core framework so you won’t need to install anything extra.
3. Do I need to dispose of Event Aggregator subscriptions?
Absolutely it’s super important to cancel any subscriptions you don’t need anymore to avoid memory leaks. Just keep in mind to use the dispose() method on those subscriptions in the detached() lifecycle method of your components.
4. Can I use Event Aggregator to communicate between parent and child components?
While you can use Event Aggregator for parent-child communication, it’s often better to use Aurelia’s binding system for direct relationships. Event Aggregator is most valuable for communication between unrelated components or distant parts of your application.
5. How do I debug Event Aggregator issues?
Here are a few simple steps to sort out any problems you might be having. First, check that the event names match up for both the publishers and subscribers. Then make sure the subscriptions are all set up before you start publishing events. Also, take a look to see if any subscriptions have been canceled too early. It could be helpful to throw in some logging to your event handlers so you can track what’s happening.
6. Can Event Aggregator work across different modules in a large Aurelia application?
Yep, the Event Aggregator works great across various parts of big Aurelia apps. Since it’s set up as a singleton in Aurelia’s dependency injection container, the same instance is used all over your app. This makes it really simple for parts in different modules to connect and communicate without any extra setups or complicated stuff.
7. What’s the difference between using string-based channels and class-based events in Aurelia’s Event Aggregator?
String-based channels, like this.ea.publish(‘user:updated’, data), are easier to set up but can cause naming clashes in bigger apps. But on the other hand, using class-based events like this.ea.publish (UserUpdatedEvent, new UserUpdatedEvent(data)) gives you better type safety and helps with autocomplete And it really simplifies keeping track of how you’re using events in your code.