How to Reverse a Linked List in Go: A Comprehensive Tutorial
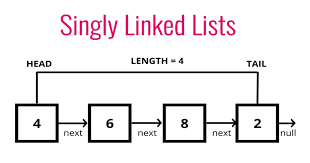
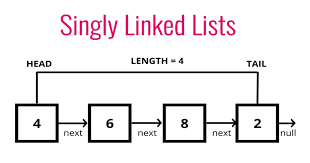
Are you looking to master one of the most common coding interview questions? In this guide, we’ll explore how to reverse a linked list using Go (Golang). We’ll cover both iterative and recursive approaches, complete with detailed explanations and performance analysis.
Understanding Linked List Reversal
Before diving into the implementation, let’s understand what reversing a linked list means. In a singly linked list, each node points to the next node in the sequence. When we reverse it, we need to make each node point to its previous node instead of its next node.
Prerequisites
- Basic understanding of Go programming
- Knowledge of linked list data structures
- Go development environment set up
Implementation Approaches
1. Iterative Solution (Three-Pointer Technique)
goCopytype Node struct {
Value int
Next *Node
}
func reverseIterative(head *Node) *Node {
var prev *Node
current := head
for current != nil {
nextTemp := current.Next
current.Next = prev
prev = current
current = nextTemp
}
return prev
}
This iterative approach has several advantages:
- Time Complexity: O(n)
- Space Complexity: O(1)
- Easy to understand and implement
- More memory efficient than recursive solution
2. Recursive Solution
goCopyfunc reverseRecursive(head *Node) *Node {
// Base case: if head is nil or we've reached the last node
if head == nil || head.Next == nil {
return head
}
// Recursive call
rest := reverseRecursive(head.Next)
// Reverse the links
head.Next.Next = head
head.Next = nil
return rest
}
Complete Working Example
Let’s see how to implement and test both solutions:
goCopypackage main
import "fmt"
type Node struct {
Value int
Next *Node
}
func printList(head *Node) {
current := head
for current != nil {
fmt.Printf("%d -> ", current.Value)
current = current.Next
}
fmt.Println("nil")
}
func main() {
// Create a sample linked list: 1->2->3->4->5
head := &Node{Value: 1}
head.Next = &Node{Value: 2}
head.Next.Next = &Node{Value: 3}
head.Next.Next.Next = &Node{Value: 4}
head.Next.Next.Next.Next = &Node{Value: 5}
fmt.Println("Original List:")
printList(head)
// Reverse using iterative method
reversed := reverseIterative(head)
fmt.Println("\nReversed List (Iterative):")
printList(reversed)
}
Performance Comparison
Let’s compare both approaches:
Iterative Solution
- Time Complexity: O(n)
- Space Complexity: O(1)
- Pros: Memory efficient, straightforward implementation
- Cons: Slightly more complex code with three pointers
Recursive Solution
- Time Complexity: O(n)
- Space Complexity: O(n) due to call stack
- Pros: Elegant and concise code
- Cons: Uses more memory due to recursive calls
Common Edge Cases and Solutions
- Empty List
goCopyif head == nil {
return nil
}
- Single Node
goCopyif head.Next == nil {
return head
}
Best Practices and Tips
- Always handle edge cases first
- Use meaningful variable names
- Test with different list lengths
- Consider memory constraints when choosing between iterative and recursive approaches
Common Interview Questions
- What is the time complexity of your solution?
- How would you handle a doubly linked list?
- Can you reverse the list in place?
- What are the trade-offs between iterative and recursive solutions?
Conclusion
Reversing a linked list is a fundamental programming challenge that tests your understanding of pointers and data structures. While both iterative and recursive solutions are valid, the iterative approach is generally preferred in production code due to its constant space complexity.
Remember to practice implementing both solutions and understanding their trade-offs. This will not only help you in coding interviews but also make you a better Go programmer.
Next Steps
- Practice implementing both solutions
- Try reversing a doubly linked list
- Implement a function to reverse only a portion of the list
- Write comprehensive test cases
FAQ
Q1: What is the most efficient way to reverse a linked list in Go?
The most efficient way to reverse a linked list in Go is using the iterative three-pointer technique. This approach has a time complexity of O(n) and a space complexity of O(1), making it more memory-efficient than the recursive solution. The iterative method uses three pointers (prev, current, and next) to track nodes while reversing the links in-place.
Q2: Can I reverse a linked list without using extra space in Go?
Yes, you can reverse a linked list without using extra space in Go using the iterative approach. The only memory used is for the three pointers (prev, current, and next), which is constant regardless of the input size. This makes the space complexity O(1). The recursive approach, while elegant, uses O(n) space due to the call stack.
Q3: How do I handle edge cases when reversing a linked list in Go?
When reversing a linked list in Go, you should handle these key edge cases:
- Empty list (head == nil): Return nil
- Single node (head.Next == nil): Return head as is
- Two nodes: Swap the pointers between them Always test your implementation with these cases to ensure robustness.
Q4: What’s the difference between reversing singly and doubly linked lists in Go?
Reversing a singly linked list requires changing each node’s Next pointer to point to the previous node. For a doubly linked list, you need to swap both Next and Prev pointers for each node. While the concept is similar, doubly linked lists require an additional step per node but can make the reversal process more straightforward since you already have access to the previous node.
Q5: How can I verify if my linked list reversal implementation is correct in Go?
To verify your linked list reversal implementation:
- Test with different list lengths (0, 1, 2, and n nodes)
- Compare the output with the expected reversed sequence
- Ensure no nodes are lost during reversal
- Check that the last node becomes the head and first node becomes the tail
- Verify that all links are properly connected by traversing the list
- Write unit tests covering all edge cases